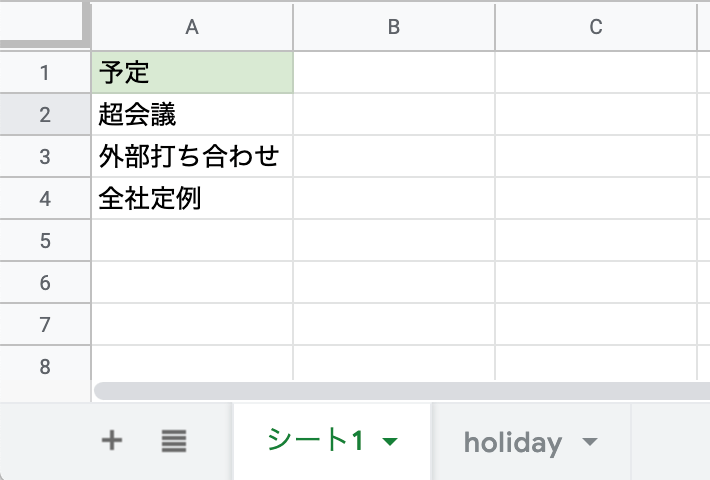
Googleカレンダーの予定を取得
GoogleスプレッドシートにGoogleカレンダーの予定を表示させるにはGoogle Apps Script (以下GAS) を使用する。
Googleスプレッドシートに自分のアカウントのGoogleカレンダーの予定を表示させたい場合は、以下のGASを保存すれば簡単に実装できる。
const email = 'example@gmail.com'
const sheetName = 'シート1'
const calender = CalendarApp.getCalendarById(email)
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(sheetName)
const schedules = calender.getEventsForDay(new Date())
function getSchedules() {
sheet.getRange("A2:A99").clearContent()
schedules.forEach((schedule, i) => {
const title = schedule.getTitle()
sheet.getRange(i + 2, 1).setValue(title)
})
}
特定の予定名だけ取得
例えば予定名に「会議」が入っている予定だけ取得したい場合はgetEventsForDayの第2引数に {search: '会議'} を付ける。
const email = 'example@gmail.com'
const sheetName = 'シート1'
const calender = CalendarApp.getCalendarById(email)
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(sheetName)
const schedules = calender.getEventsForDay(new Date(), {search: '会議'})
function getSchedules() {
sheet.getRange("A2:A99").clearContent()
schedules.forEach((schedule, i) => {
const title = schedule.getTitle()
sheet.getRange(i + 2, 1).setValue(title)
})
}
ただし、searchオプションだと否定や正規表現による判定ができないので、schedule.getTitle()をtestメソッドで判定したほうが良いだろう。
function getSchedules() {
sheet.getRange("A2:A99").clearContent()
let i = 2
schedules.forEach(schedule => {
const title = schedule.getTitle()
if (!/会議/.test(title)) {
sheet.getRange(i++, 1).setValue(title)
}
})
}
不参加の予定名は取得しない
Googleカレンダーの予定は参加・不参加を登録できいるが、不参加の予定は取得したくない場合が多い。
そんなときはgetMyStatus()で不参加(NO)を取得できるので、これで判定して除外すればよい。
function getSchedules() {
sheet.getRange("A2:A99").clearContent()
let i = 2
schedules.forEach(schedule => {
const title = schedule.getTitle()
const myStatus = schedule.getMyStatus()
if (!/NO/.test(myStatus)) {
sheet.getRange(i++, 1).setValue(title)
}
})
}
土・日は取得しない
月曜から金曜の予定は取得したいが、土・日の場合は月曜から取得したい場合がある。
そんなときはgetDate()で6, 7のときにsetDate()で2, 1をセットして月曜にする。
const email = 'example@gmail.com'
const sheetName = 'シート1'
const calender = CalendarApp.getCalendarById(email)
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(sheetName)
const now = new Date()
const day = now.getDay()
let addDate = 0
if (day === 6) addDate = 2
if (day === 7) addDate = 1
now.setDate(now.getDate() + addDate)
const schedules = calender.getEventsForDay(now)
function getSchedules() {
sheet.getRange("A2:A99").clearContent()
schedules.forEach((schedule, i) => {
const title = schedule.getTitle()
sheet.getRange(i + 2, 1).setValue(title)
})
}
土・日・祝日は取得しない
土・日だけでなく祝日も取得しない場合はholidayシートを作成してその中に祝日の日付を入れて、new Date()がholidayシート内の日付と一致するかどうかでsetDateを追加すれば良い。
祝日一覧は内閣府発行のCSVファイルを使用する。
CSVではなく祝日カレンダー(ja.japanese#holiday@group.v.calendar.google.com)から祝日をgetEventsForDayで判定することもできるが、その場合は祝日一覧に有給休暇などを追加して運用することなどができなくなる。
const email = 'example@gmail.com'
const sheetName = 'シート1'
const calender = CalendarApp.getCalendarById(email)
const ss = SpreadsheetApp.getActiveSpreadsheet()
const sheet = ss.getSheetByName(sheetName)
const sheetHoliday = ss.getSheetByName('holiday')
const now = new Date()
const day = now.getDay()
let addDate = 0
if (day === 6) addDate = 2
if (day === 7) addDate = 1
now.setDate(now.getDate() + addDate)
const holidays = sheetHoliday.getRange('A2:A30').getValues()
holidays.forEach(holiday => {
var isDateEqual = (new Date(holiday[0]).getTime() === new Date(now.getFullYear(), now.getMonth(), now.getDate()).getTime())
if (isDateEqual) {
now.setDate(now.getDate() + 1)
} else if (now.getDay() === 6) {
now.setDate(now.getDate() + 2)
}
})
const schedules = calender.getEventsForDay(now)
function getSchedules() {
sheet.getRange("A2:A99").clearContent()
let i = 2
schedules.forEach(schedule => {
const title = schedule.getTitle()
sheet.getRange(i++, 1).setValue(title)
})
}
時間や説明文なども取得可能
サンプルのコードでは予定名だけをgetTitle()で取得しているが、時間や説明文なども以下のメソッドを使用すれば取得可能。
メソッド | 説明 |
---|---|
getAllDayEndDate() | Gets the date on which this all-day calendar event ends. |
getAllDayStartDate() | Gets the date on which this all-day calendar event begins. |
getAllTagKeys() | Gets all keys for tags that have been set on the event. |
getColor() | Returns the color of the calendar event. |
getCreators() | Gets the creators of the event. |
getDateCreated() | Gets the date the event was created. |
getDescription() | Gets the description of the event. |
getEmailReminders() | Gets the minute values for all email reminders for the event. |
getEndTime() | Gets the date and time at which this calendar event ends. |
getEventSeries() | Gets the series of recurring events that this event belongs to. |
getGuestByEmail( | Gets a guest by email address. |
getGuestList() | Gets the guests for the event, not including the event owner. |
getGuestList( | Gets the guests for the event, potentially including the event owners. |
getId() | Gets the unique iCalUID of the event. |
getLastUpdated() | Gets the date the event was last updated. |
getLocation() | Gets the location of the event. |
getMyStatus() | Gets the event status (attending, etc.) of the effective user. |
getOriginalCalendarId() | Get the ID of the calendar where this event was originally created. |
getPopupReminders() | Gets the minute values for all popup reminders for the event. |
getSmsReminders() | Gets the minute values for all SMS reminders for the event. |
getStartTime() | Gets the date and time at which this calendar event begins. |
getTag( | Gets a tag value of the event. |
getTitle() | Gets the title of the event. |
getVisibility() | Gets the visibility of the event. |