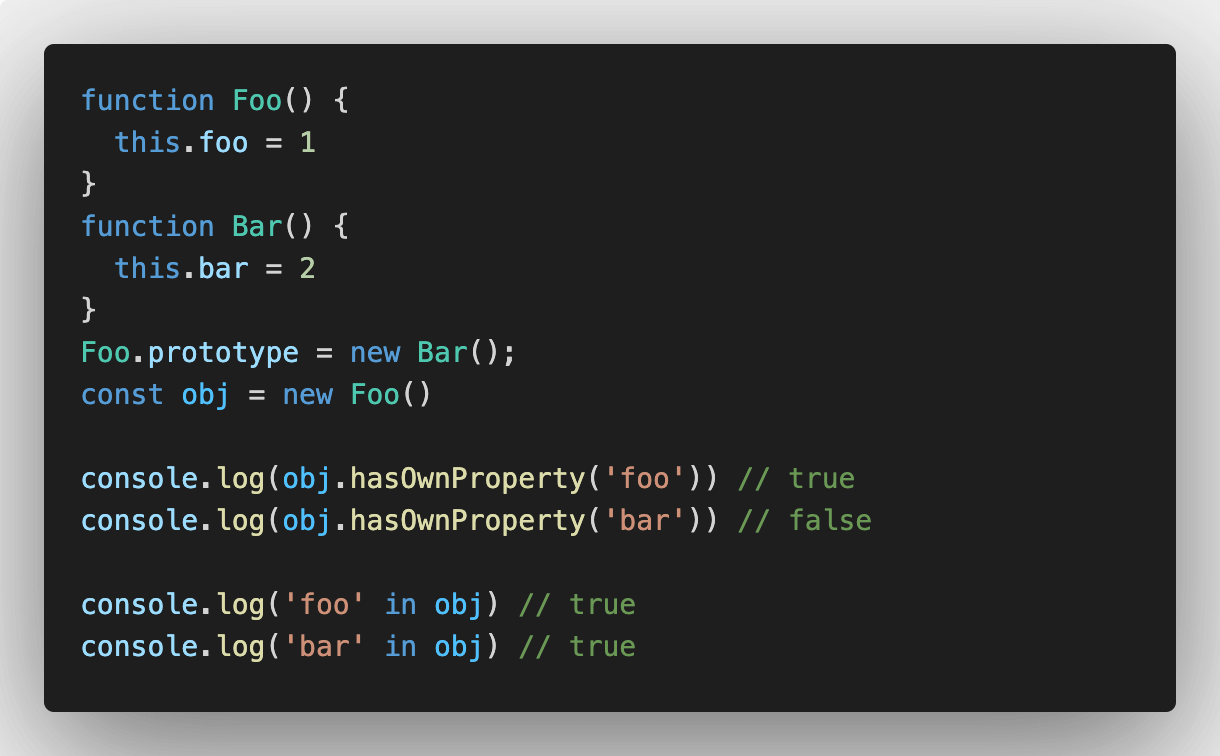
Objectのin演算子とは
JavaScriptのオブジェクトのプロパティの有無を確認できる演算子。
オブジェクトのプロパティの有無は古い書き方だとObject.prototype.hasOwnProperty.callを使う方法があるが、in演算子なら以下のように短い記述でプロパティの有無を確認できる。
const foo = {
example: 123
}
console.log(Object.prototype.hasOwnProperty.call(foo, 'sample')) // false
console.log(Object.prototype.hasOwnProperty.call(foo, 'example')) // true
console.log('sample' in foo) // false
console.log('example' in foo) // true
ES2022からObject.hasOwn()メソッドが追加されているので演算子ではなくメソッドで確認したい場合はこちらを使う選択肢もある。
const foo = {
example: 123
}
console.log(Object.hasOwn(foo, 'sample')) // false
console.log(Object.hasOwn(foo, 'example')) // true
オブジェクトにhasOwnProperty()を直接付けて確認しているコードもたまに見かけるが、prototypeチェーンの元のプロパティを見て判定できないので注意が必要。
function Foo() {
this.foo = 1
}
function Bar() {
this.bar = 2
}
Foo.prototype = new Bar()
const obj = new Foo()
console.log(obj.hasOwnProperty('foo')) // true
console.log(obj.hasOwnProperty('bar')) // false
console.log('foo' in obj) // true
console.log('bar' in obj) // true