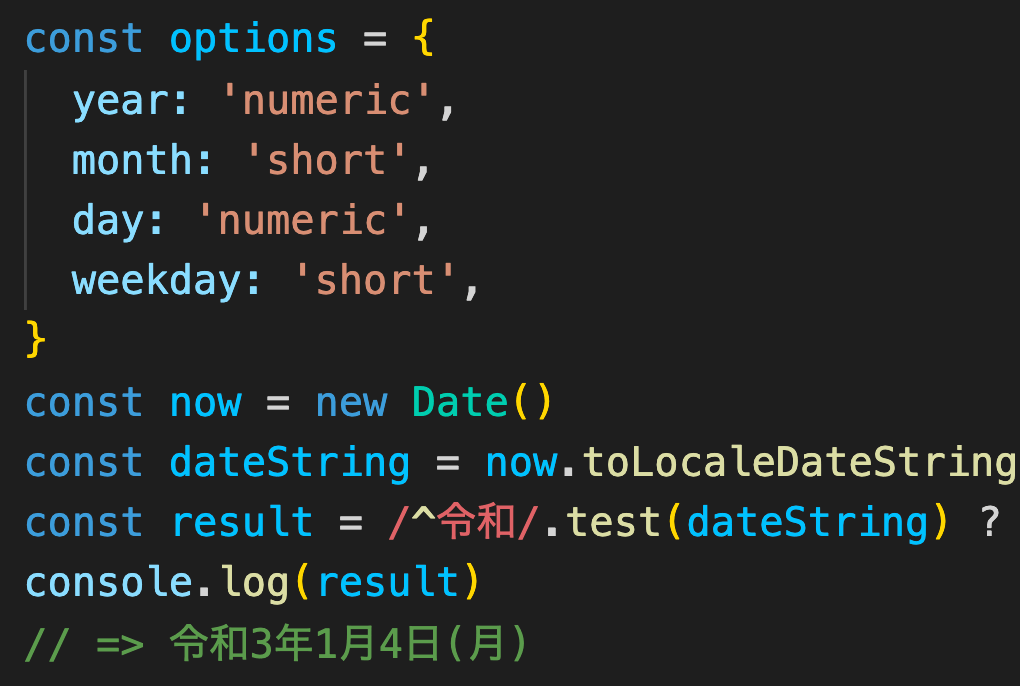
getMonthなどを使わないで日付表示
JavaScriptで2020年1月4日(月)のような日付を表示するにはnew Date()で日付を取得して、getFullYear, getMonth, getDate, getDayを使用して以下のようにして作成すると学習する人が多い。
const now = new Date()
const year = now.getFullYear()
const month = now.getMonth() + 1
const date = now.getDate()
const day = '日月火水木金土'.charAt(now.getDay())
console.log(`${year}年${month}月${date}日(${day})`)
// => 2021年1月4日(月)
しかし、getFullYear, getMonth, getDate, getDayを使用しなくてもtoLocaleDateString()を使用すれば同じ文字列を作成することができる。
toLocaleDateString()の日付作成
toLocaleDateString()を使用する場合はnew Date()を作成するのは同じで、ここに.toLocaleDateString('ja-JP')を付ける。
const now = new Date()
const dateString = now.toLocaleDateString('ja-JP')
console.log(dateString)
// => 2021/1/4
これだけだと年や月はスラッシュとなり曜日も付かない。
年、月、曜日を付けるにはtoLocaleDateStringの第2引数に以下のようなオプションを付ける。
const options = {
year: 'numeric',
month: 'short',
day: 'numeric',
weekday: 'short',
}
const now = new Date()
const dateString = now.toLocaleDateString('ja-JP', options)
console.log(dateString)
// => 2021年1月4日(月)
このオプションはyearがなければ2021年の部分は表示されず、さらにweekdayがなければ曜日が表示されない。
const options = {
month: 'short',
day: 'numeric',
}
const now = new Date()
const dateString = now.toLocaleDateString('ja-JP', options)
console.log(dateString)
// => 1月4日
ja-JP-u-ca-japaneseで元号表示
toLocaleDateStringの第1引数にja-JPではなくja-JP-u-ca-japaneseを指定すると日付が元号で表示できる。
ただし、一部の古いブラウザだと令和3年と表示するべきところを3年とだけ表示してしまうので令和が付いていなければ追加する処理も必要。
const options = {
year: 'numeric',
month: 'short',
day: 'numeric',
weekday: 'short',
}
const now = new Date()
const dateString = now.toLocaleDateString('ja-JP-u-ca-japanese', options)
const result = /^令和/.test(dateString) ? dateString : '令和' + dateString
console.log(result)
// => 令和3年1月4日(月)