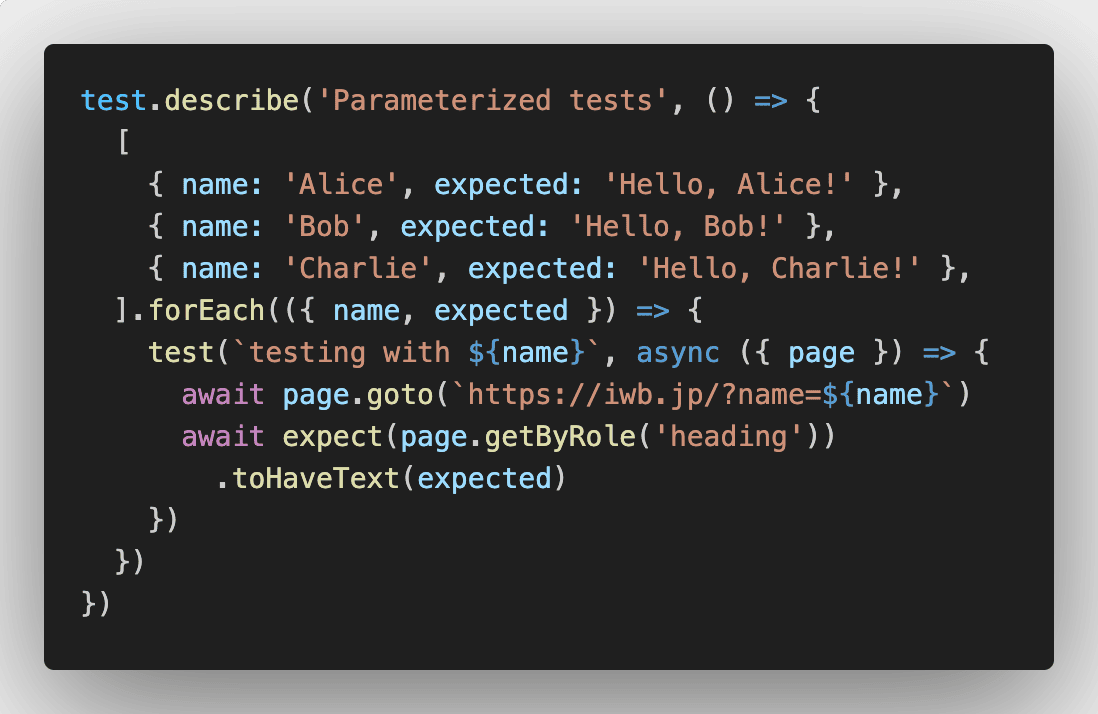
Parameterized testsとは
以下のコードのように配列でパラメーターを用意して、forEachなどのループを使用したテストです。
example.spec.ts
[
{ name: 'Alice', expected: 'Hello, Alice!' },
{ name: 'Bob', expected: 'Hello, Bob!' },
{ name: 'Charlie', expected: 'Hello, Charlie!' },
].forEach(({ name, expected }) => {
// You can also do it with test.describe() or with multiple tests as long the test name is unique.
test(`testing with ${name}`, async ({ page }) => {
await page.goto(`https://example.com/greet?name=${name}`);
await expect(page.getByRole('heading')).toHaveText(expected);
});
});
https://playwright.dev/docs/test-parameterize
これは公式のテストコードですが、すでにtest()が書かれているコードに追記すると、「Left side of comma operator is unused and has no side effects.」というエラーが表示されます。
配列を変数に入れればエラーは表示されなくなります。
example.spec.ts
import { test, expect } from '@playwright/test'
const data = [
{ name: 'Alice', expected: 'Hello, Alice!' },
{ name: 'Bob', expected: 'Hello, Bob!' },
{ name: 'Charlie', expected: 'Hello, Charlie!' },
]
data.forEach(({ name, expected }) => {
test(`testing with ${name}`, async ({ page }) => {
await page.goto(`https://example.com/greet?name=${name}`)
await expect(page.getByRole('heading')).toHaveText(expected)
})
})
しかし、これだとtest()の開始位置がわかりづらくなるので、test.describe()で囲んだほうがスマートです。
example.spec.ts
import { test, expect } from '@playwright/test'
test.describe('Parameterized tests', () => {
[
{ name: 'Alice', expected: 'Hello, Alice!' },
{ name: 'Bob', expected: 'Hello, Bob!' },
{ name: 'Charlie', expected: 'Hello, Charlie!' },
].forEach(({ name, expected }) => {
test(`testing with ${name}`, async ({ page }) => {
await page.goto(`https://example.com/greet?name=${name}`)
await expect(page.getByRole('heading')).toHaveText(expected)
})
})
})
example.spec.tsのコードはエラーになる
この公式のテストコードは実行してもh1の「page.getByRole('heading')」の値は変わらないのでエラーになります。
以下のテストコードであればテストをパスします。
example.spec.ts
import { test, expect } from '@playwright/test'
test.describe('Parameterized tests', () => {
[
{ name: 'Alice', expected: 'Hello, Alice!' },
{ name: 'Bob', expected: 'Hello, Bob!' },
{ name: 'Charlie', expected: 'Hello, Charlie!' },
].forEach(({ name, expected }) => {
test(`testing with ${name}`, async ({ page }) => {
await page.goto(`https://iwb.jp/s/playwright-parameterized-tests/?name=${name}`)
await expect(page.getByRole('heading')).toHaveText(expected)
})
})
})
リンク先のWebページはテストがパスするように以下のようになっています。
HTML
<h1>Hello, <span id="name"></span>!</h1>
<script>
const url = new URL(window.location.href)
const params = url.searchParams
const paramsName = params.get('name')
document.getElementById('name').textContent = paramsName
</script>
このコードなら大半の方はParameterized testsを理解することができると思います。