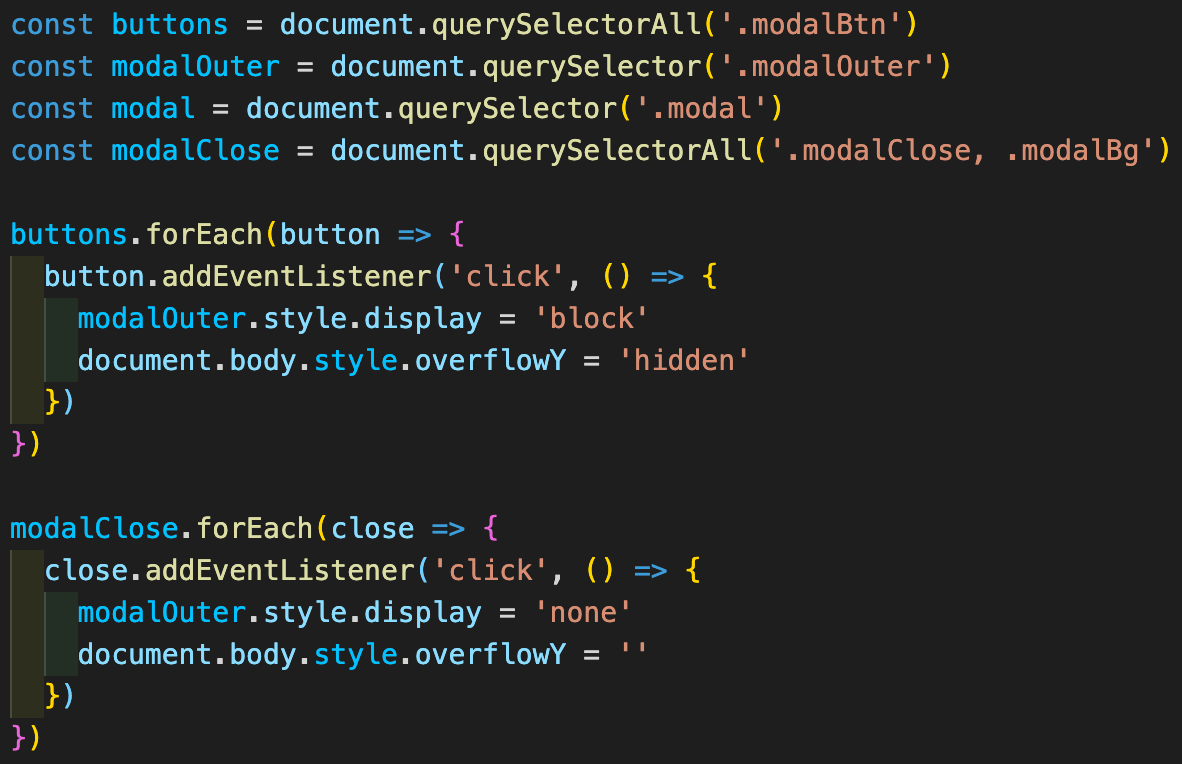
1KB以下のJSでモーダル作成
初心者の方だとJavaScriptでモーダルの処理を書くのは難しいと思われがちだが、必要最小限のコードで書けば簡単な処理を18行程度記述するだけで実装できる。
HTMLの用意
まず以下のようなモーダル用のHTMLを用意する。
テキストなどは.modalInner内に入れる。
<div class="modalOuter">
<div class="modal">
<div class="modalClose">✖</div>
<div class="modalInner">
<p>Sample Modal</p>
</div>
</div>
<div class="modalBg"></div>
</div>
開くためのボタンのHTMLは以下のようになっているものとする。
<button class="modalBtn">Show Modal</button>
CSSの用意
まず以下のCSSに追加する。
Reset CSSなどですでに適用されているWebサイトであれば以下の記述は不要。
*, *::before, *::after {
box-sizing: border-box;
word-break: break-all;
}
html, body {
height: 100%;
}
次にモーダル用のCSSを用意する。
モーダルは最初は非表示状態にする必要があるため.modalOuterは非表示にする。
.modalOuter {
display: none;
}
.modalは位置を固定するためposition: fixed;を指定。
SPとPCのどちらのWebページで使用できるようwidthは%で指定。
幅が大きくなりすぎないようにmax-widthも指定しておく。
.modal {
position: fixed;
top: 50%;
left: 50%;
z-index: 99999;
transform: translate(-50%, -50%);
width: 90%;
max-width: 400px;
height: 300px;
border: 1px solid #000;
box-shadow: 0 0 5px rgba(0, 0, 0, 0.5);
background: #fff;
}
.modalInnerはテキストなどが入る箇所だが指定した高さを超えてもはみ出さないようoverflow-y: scroll;を必ず指定する。
height: inherit;を指定して.modalからheight値を継承するのも忘れずに。
.modalInner {
overflow-y: scroll;
height: inherit;
padding: 1rem;
}
.modalBgはモーダルの透過背景部分なので、.modalと同様にposition: fixed;で固定する。
.modalBg {
position: fixed;
top: 0;
left: 0;
z-index: 9999;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.7);
}
.modalCloseは閉じるボタンなのでposition: absolute;を指定して適切な場所に配置すれば、あとのスタイルは適当で良い。(下記の例と同じである必要はない)
.modalClose {
display: grid;
place-content: center;
position: absolute;
top: -15px;
right: -15px;
width: 40px;
height: 40px;
border: 2px solid #000;
border-radius: 50%;
background: #fff;
cursor: pointer;
}
JavaScriptの用意
最後にJavaScriptを用意するのだが、「モーダルを表示する処理」と「モーダルを閉じる処理」の2つがあれば良いので、以下のように簡単な処理で実装することができる。
buttonsの要素がクリックされた際に表示し、modalCloseがクリックされたときに閉じる。
モーダルを表示するだけだと開いている最中にWebページがスクロールできてしまうのでbodyタグにoverflow-y: hidden;が追加されるようにしてある。
const buttons = document.querySelectorAll('.modalBtn')
const modalOuter = document.querySelector('.modalOuter')
const modal = document.querySelector('.modal')
const modalClose = document.querySelectorAll('.modalClose, .modalBg')
buttons.forEach(button => {
button.addEventListener('click', () => {
modalOuter.style.display = 'block'
document.body.style.overflowY = 'hidden'
})
})
modalClose.forEach(close => {
close.addEventListener('click', () => {
modalOuter.style.display = 'none'
document.body.style.overflowY = ''
})
})