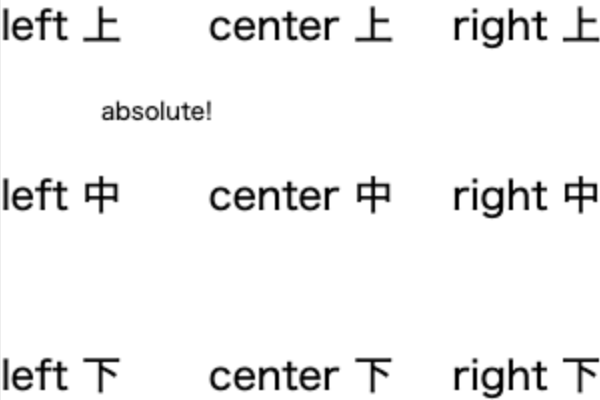
Canvasテキスト配置関数
HTML+CSSならテキスト配置は現在はdisplay: flexなどの豊富なプロパティが使用できるので比較的簡単に実装可能だ。
しかし、Canvasだと位置調整がかなり面倒なので簡単にテキストの配置ができる関数を作成してみた。
Canvasテキスト配置関数 使い方
サンプル作成のため、まず以下の基本的なHTML, CSSを記述する。
<canvas id="canvas"></canvas>
body { background: #eee; } #content { width: 300px; margin: 0 auto; }
そしてcanvasを300 x 200で作成。
const canvas = document.getElementById('canvas') const ctx = canvas.getContext('2d') const ctxWidth = 300 const ctxHeight = 200 canvas.width = ctxWidth canvas.height = ctxHeight canvas.style.background = '#fff'
そして次に関数を記述。
function ctxFillText(text, fontSize, textX, textY, fontStyle, fontFamily) { ctx.font = ( fontStyle ? fontStyle : 'normal') + ' ' + fontSize + 'px ' + (fontFamily ? fontFamily : 'sans-serif') ctx.fillText( text, textX === 'center' ? (ctxWidth - ctx.measureText(text).width) / 2 : textX === 'right' ? ctxWidth - ctx.measureText(text).width: textX && textX !== 'left' ? textX : 0, textY === 'center' ? (ctxHeight + fontSize / 2) / 2 : textY === 'bottom' ? ctxHeight - fontSize / 4 : textY && textY !== 'top' ? textY : fontSize ) }
使い方は以下のように基本は第1から第4引数にテキスト、フォントサイズ、X位置、Y位置を入力するだけだ。
textX, textYが数値なら絶対配置。'center'などを指定している場合は横位置(縦位置)で中央揃えを行う。
これらを使用すればcanvasでのテキスト配置が直感的に行えるようになるだろう。
// ctxFillText(text, fontSize, textX, textY) ctxFillText('absolute!', 12, 50, 60) // 'left', 'top' is default ctxFillText('left 上', 20) ctxFillText('left 中', 20, null, 'center') ctxFillText('left 下', 20, null, 'bottom') ctxFillText('center 上', 20, 'center', 'top') ctxFillText('center 中', 20, 'center', 'center') ctxFillText('center 下', 20, 'center', 'bottom') ctxFillText('right 上', 20, 'right', 'top') ctxFillText('right 中', 20, 'right', 'center') ctxFillText('right 下', 20, 'right', 'bottom')