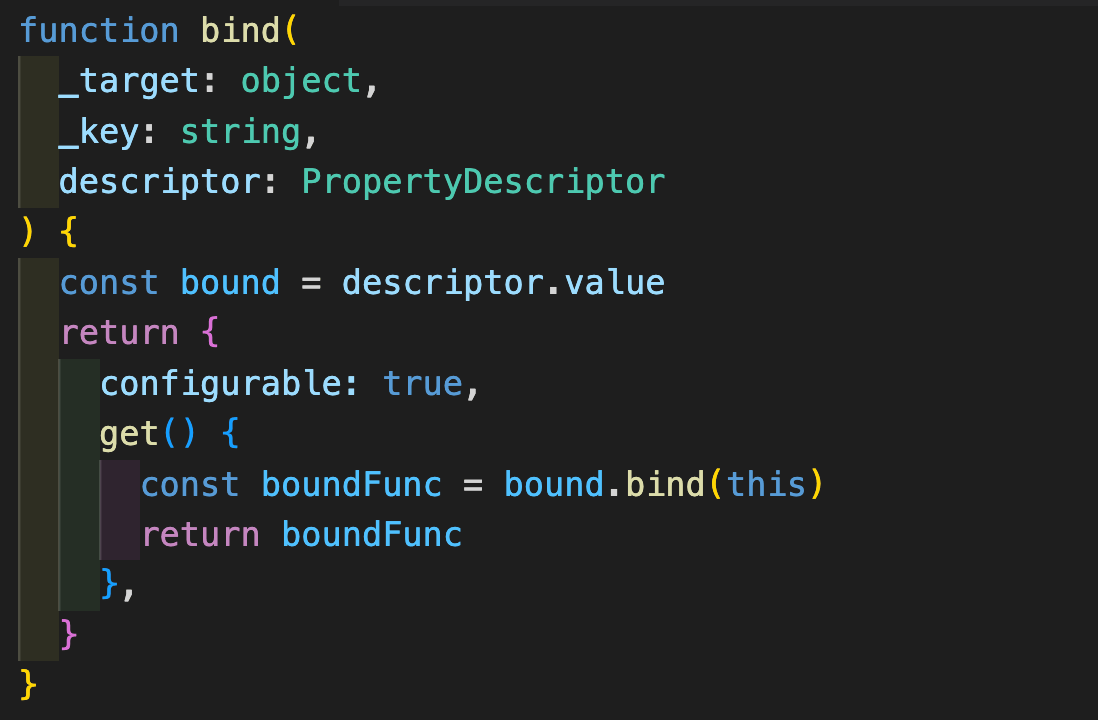
.bind(this)用デコレーターをnpm installで実装
TypeScriptを使用してWebサイトを作成するときに.bind(this)用デコレーターが作成されていることがある。
.bind(this)用デコレーターとは以下のようなコードのやつです。
function bind(
_target: object,
_key: string,
descriptor: PropertyDescriptor
) {
const bound = descriptor.value
return {
configurable: true,
get() {
const boundFunc = bound.bind(this)
return boundFunc
},
}
}
class Component {
// 省略
@bind
private submit(event: Event) {
event.preventDefault()
console.log(this.mail.value)
}
}
.bind(this)用デコレーターを利用すればフォームの要素を取得するための.bind(this)を何回も書かなくて済みますが、書き方が人によって異なるというデメリットがあります。
npmにはすでに.bind(this)用デコレーターが用意されているので、autobind decoratorを利用すれば5秒で実装可能です。
autobind decoratorの使い方
まず以下のコマンドでインストールします。
npm i -D autobind-decorator
インストール完了後は以下のようにimportすれば使用可能です。
import { boundMethod } from 'autobind-decorator'
class Component {
constructor(value) {
this.value = value
}
@boundMethod
method() {
return this.value
}
}
const component = new Component(7)
const method = component.method
method() // 7
ちなみにbind-decoratorというのもあるのですが、こちらは開発時期が古くてimport時の名前がbindであるなどのせいでautobind-decoratorほど利用されていないようです。