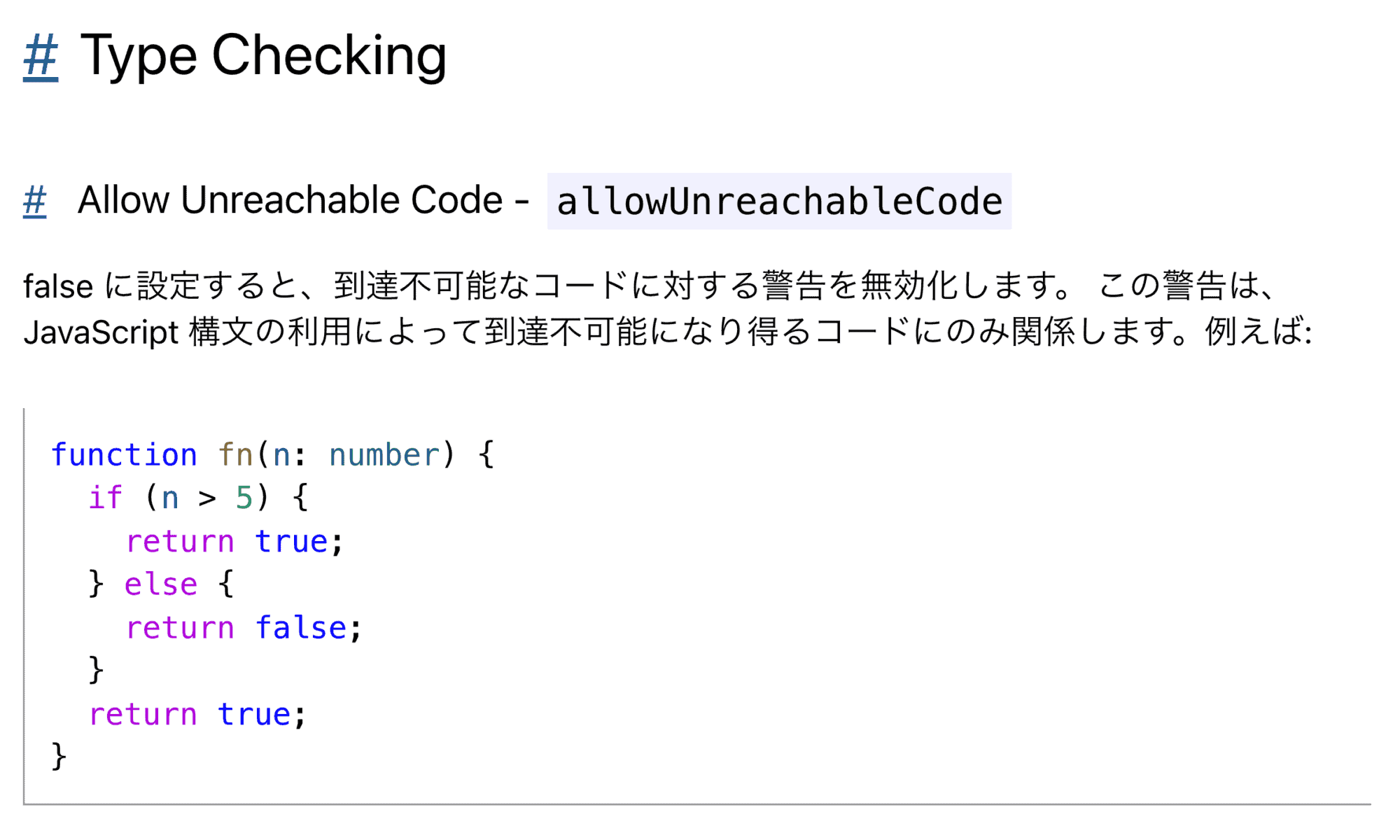
tsconfig.jsonの初期設定
TypeScriptでtsconfig.jsonを用意する際は「npx tsc --init」で生成するか、Viteで環境構築時に生成することが多いです。
npx tsc --initで生成したtsconfig.json (コメント除去済み)
{
"compilerOptions": {
"target": "es2016",
"module": "commonjs",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true
}
}
Vite(vanilla-ts)で生成したtsconfig.json
npm create vite@latest my-vite-ts -- --template vanilla-ts
{
"compilerOptions": {
"target": "ES2020",
"useDefineForClassFields": true,
"module": "ESNext",
"lib": ["ES2020", "DOM", "DOM.Iterable"],
"skipLibCheck": true,
/* Bundler mode */
"moduleResolution": "bundler",
"allowImportingTsExtensions": true,
"resolveJsonModule": true,
"isolatedModules": true,
"noEmit": true,
/* Linting */
"strict": true,
"noUnusedLocals": true,
"noUnusedParameters": true,
"noFallthroughCasesInSwitch": true
},
"include": ["src"]
}
見ての通り、npx tsc --initでは「strict」、Vite(vanilla-ts)のほうは「strict, noUnusedLocals, noUnusedParameters, noFallthroughCasesInSwitch」のLintingがtrueで有効化されています。
strictは以下の8個をすべて有効にするオプションです。
- alwaysStrict
- noImplicitAny
- noImplicitThis
- strictNullChecks
- strictFunctionTypes
- strictBindCallApply
- strictPropertyInitialization
- useUnknownInCatchVariables
Viteではstrict以外にも以下の3個も有効になっています。
- noUnusedLocals
- noUnusedParameters
- noFallthroughCasesInSwitch
この3個は使用していない変数、パラメータ、switchのcaseなどがあるときに警告されます。
これらはコードの記述ミスを防ぐために有効なので、必ず設定することをオススメします。
tsconfig.jsonのオプションは全部で19個
tsconfig.jsonのLinterのオプションは全部で19個存在します。(2024年4月現在)
オプションの一覧とVite(vanilla-ts)で設定されているものは以下の通りです。
Option | Vite |
---|---|
allowUnreachableCode | |
allowUnusedLabels | |
alwaysStrict | ✅ |
exactOptionalPropertyTypes | |
noFallthroughCasesInSwitch | ✅ |
noImplicitAny | ✅ |
noImplicitOverride | |
noImplicitReturns | |
noImplicitThis | ✅ |
noPropertyAccessFromIndexSignature | |
noUncheckedIndexedAccess | |
noUnusedLocals | ✅ |
noUnusedParameters | ✅ |
strict | ✅ |
strictBindCallApply | ✅ |
strictFunctionTypes | ✅ |
strictNullChecks | ✅ |
strictPropertyInitialization | ✅ |
useUnknownInCatchVariables | ✅ |
見ての通り、Viteでは19個のうち12個のLinterのオプションが使用されており、以下の7個が未使用になっています。
- allowUnreachableCode
- allowUnusedLabels
- exactOptionalPropertyTypes
- noImplicitOverride
- noImplicitReturns
- noPropertyAccessFromIndexSignature
- noUncheckedIndexedAccess
これら未使用の7個のオプションも有効にしておけば、よりバグの少ないコードが書ける可能性があります。
ちなみに19個すべてのオプションを有効にする場合は以下のようになります。
{
"compilerOptions": {
"strict": true,
"noUnusedLocals": true,
"noUnusedParameters": true,
"noFallthroughCasesInSwitch": true,
"allowUnreachableCode": true,
"allowUnusedLabels": true,
"exactOptionalPropertyTypes": true,
"noImplicitOverride": true,
"noImplicitReturns": true,
"noPropertyAccessFromIndexSignature": true,
"noUncheckedIndexedAccess": true
}
}
strictはalwaysStrictなどの8個のオプションをすべて有効にするオプションなので、strictを使わずに以下のように書くこともできます。
strictの中にfalseにしたいオプションがある場合に有効な書き方です。
{
"compilerOptions": {
"alwaysStrict": true,
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": true,
"strictFunctionTypes": true,
"strictBindCallApply": true,
"strictPropertyInitialization": true,
"useUnknownInCatchVariables": true,
"noUnusedLocals": true,
"noUnusedParameters": true,
"noFallthroughCasesInSwitch": true,
"allowUnreachableCode": true,
"allowUnusedLabels": true,
"exactOptionalPropertyTypes": true,
"noImplicitOverride": true,
"noImplicitReturns": true,
"noPropertyAccessFromIndexSignature": true,
"noUncheckedIndexedAccess": true
}
}
tsconfig.jsonを初期設定のままにせず、これらも本当に必要がないか、調べた上で設定することをオススメします。