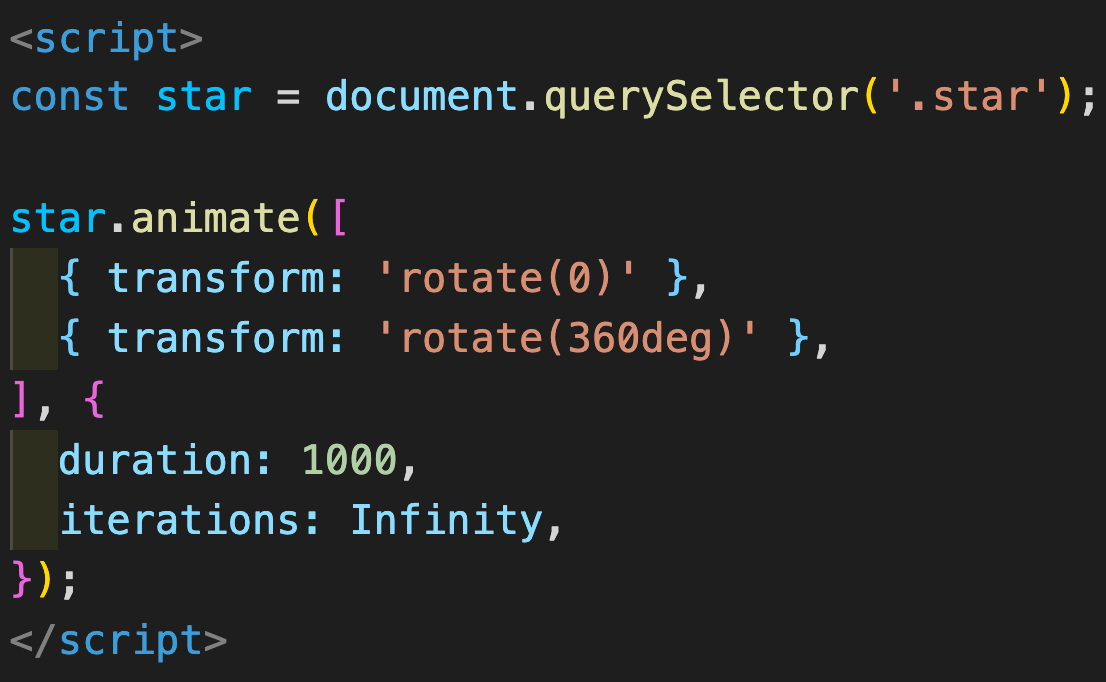
CSSなしでアニメーション
特定の要素に単純なアニメーションを追加する場合、CSSが使用されることが多い。
例えば下記の⭐️を回転させる場合、以下のようにCSSを記述して動かす。
.star {
display: inline-block;
font-size: 3em;
animation: rotate 1s linear infinite;
}
@keyframes rotate {
to {
transform: rotate(360deg);
}
}
⭐️
JavaScriptのWeb Animations APIを使用するとCSSなしで同様にアニメーションを追加させることができる。
CSSと同様に開始のrotate(0)は省略可能。
star.animate([
{ transform: 'rotate(360deg)' },
], {
duration: 1000,
iterations: Infinity,
});
プロパティが複数ある場合は以下のように記述する。
star.animate([
{ transform: 'rotate(360deg) translate3D(-50%, -50%, 0)', fontSize: '6rem' },
], {
duration: 1000,
iterations: Infinity,
});
JavaScriptだとif文を入れられるのでCSSのアニメーションと異なり特定の条件により動きを変えることもできる。
Web Animations APIの使い方
基本的な使い方はCSSアニメーションのようにプロパティ、値、所要時間、繰り返し回数などを記述するだけ。
@keyframesの0%, 20%, 100%のような指定はoffsetで0〜1を指定することで実装できる。
/* CSSの場合 */
@keyframes rotate {
0% {
transform: rotate(0deg);
}
20% {
transform: rotate(180deg);
}
100% {
transform: rotate(360deg);
}
}
// JavaScriptの場合
const star = document.querySelector('.star');
star.animate([
{ transform: 'rotate(0)' },
{ transform: 'rotate(180deg)', offset: 0.2 },
{ transform: 'rotate(360deg)' },
], {
duration: 1000,
iterations: Infinity,
});
easingで速度の変化を追加
CSSアニメーションと同様にeasingを設定できる。
const star = document.querySelector('.star');
star.animate([
{ transform: 'rotate(360deg)' },
], {
duration: 1000,
iterations: Infinity,
easing: 'ease-in-out',
});
ちなみにeasingでstepsも指定できる。
star.animate([
{ transform: 'rotate(360deg)' },
], {
duration: 1000,
iterations: Infinity,
easing: 'steps(3, end)',
});
アニメーションの一時停止と再生
一時停止と再生はpause()およびplay()を実行するだけでできる。
const star = document.querySelector('.star');
const button = document.getElementById('button');
const starAnime = star.animate([
{ transform: 'rotate(360deg)' },
], {
duration: 1000,
iterations: Infinity,
});
button.addEventListener('click', () => {
starAnime.playState === 'running' ?
starAnime.pause() :
starAnime.play();
});
Web Animations API 一時停止と再生サンプル
始点に戻らないようfill: 'forwards'を設定
CSSアニメーションと同様にforwardsが設定されていないとアニメーション終了時に開始位置に戻ってしまうので開始位置に戻さない場合はfill: 'forwards'を設定する必要がある。
const ball = document.querySelector('.ball');
ball.animate([
{ transform: 'translateX(200px)' },
], {
duration: 1000,
fill: 'forwards',
});
参考
Web Animations API を利用する - Web API | MDN