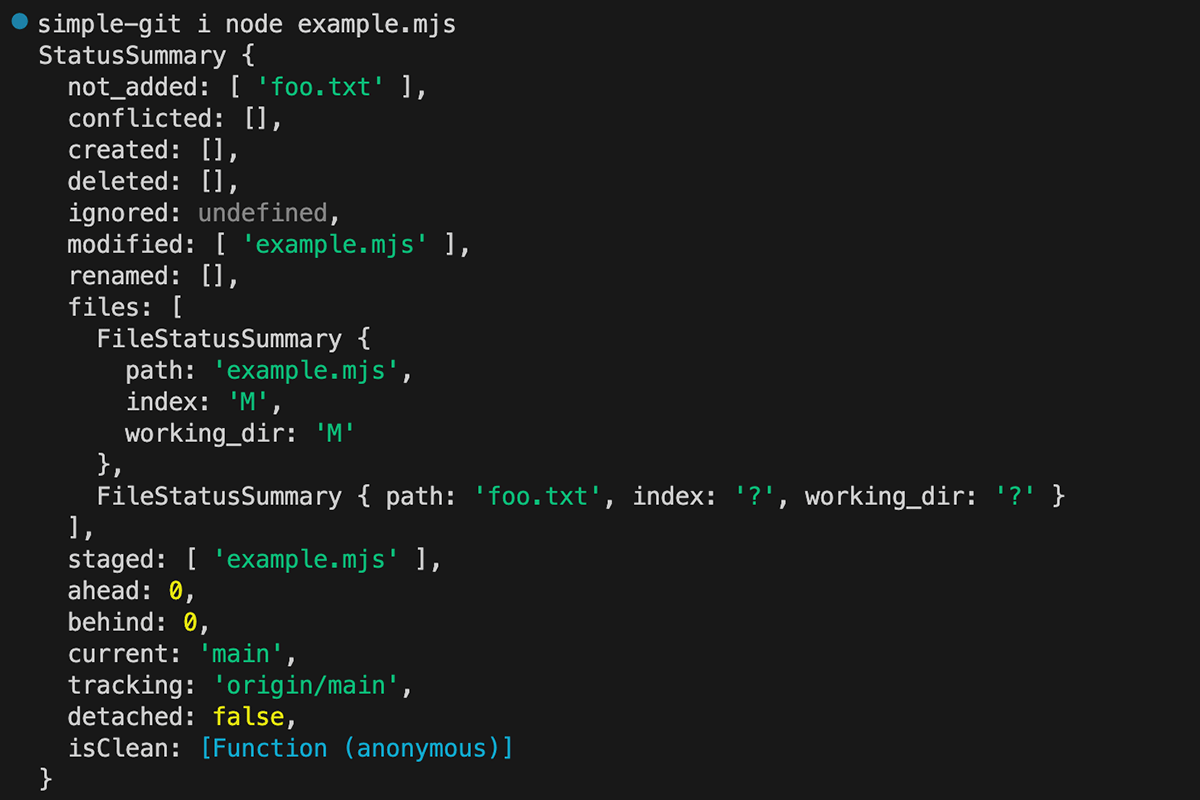
Node.jsでGitを使用する
Gitのコミットなどの各コマンドは通常はターミナル上で直接入力して実行しますが、Node.jsを使用してコミットなどの各コマンドを実行したいことがあります。
そんなときはsimple-gitを使用すれば簡単にNode.jsでもGitの各コマンドを実行できます。
simple-gitは以下のコマンドでインストールしてください。
npm i -D simple-git
simple-gitの使い方
import { simpleGit } from 'simple-git' でsimpleGitをimportして各Gitコマンドのメソッドを使用します。
例えば「git status」の場合は以下のようになります。
// example.mjs
import { simpleGit } from 'simple-git'
simpleGit()
.status()
.then((status) => console.log(status))
example.mjsを作成したら「node example.mjs」を実行するとconsole.logでstatusが見られます。
ターミナルのgit statusを実行したときと異なり、オブジェクトで下図のように返されます。
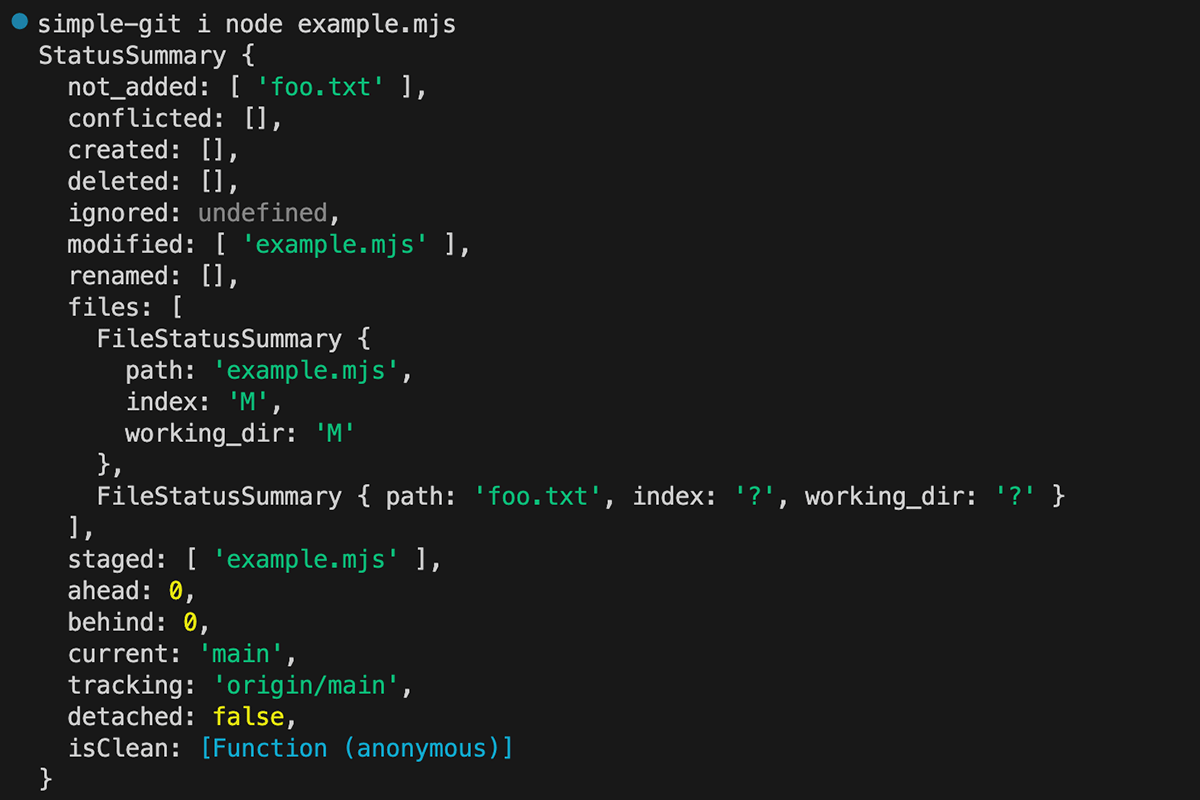
オブジェクトでgit statusが返されるので、simpleGit().status() のmodifiedだけのaddも簡単にできます。
simpleGit()
.status()
.then((status) => {
simpleGit().add(status.modified)
console.log(status)
})
もちろんbranchの作成なども可能です。
Node.jsを使用しているので、ブランチ名に作成日の日付を付けるみたいなブランチの作成もできます。
import { simpleGit } from 'simple-git'
const today = new Date()
const year = today.getFullYear()
const month = String(today.getMonth() + 1).padStart(2, '0')
const day = String(today.getDate()).padStart(2, '0')
simpleGit().checkoutLocalBranch(`feature/${year}${month}${day}`)
// feature/20230607 のようなブランチが作成されます
ほかにも git.pull() や git.push() などの基本コマンドはsimple-gitでも使用可能なので、詳しくはsimple-gitの公式サイト(GitHub)をご確認ください。
https://github.com/steveukx/git-js