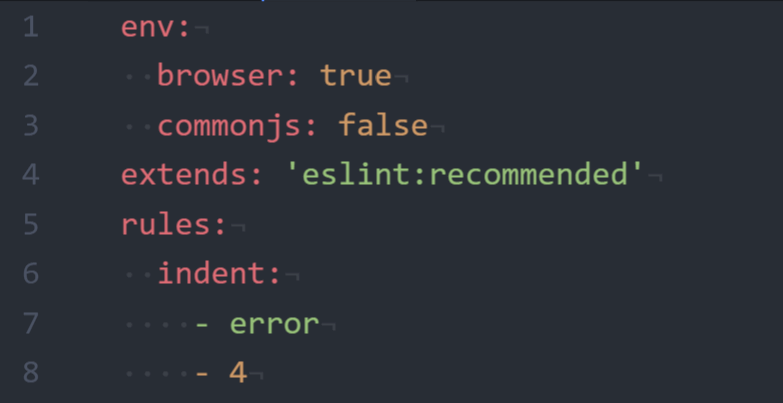
目次
ESLintとは
JavaScriptのコードが正しいかチェックするツール。
以下の手順で簡単にインストールして使用可能。
バージョンは6.8.0のものなので使用バージョンによっては質問内容が異なる場合がある。
1. npmをインストールする
2. ターミナルを開く
ターミナル(コマンドプロンプト)でESLintをインストールするディレクトリに移動
cd ディレクトリのパス
3. ESLintをインストールする
npm i -g eslint
または
sudo npm i -g eslint
4. ESLintの初期設定を行う
eslint --init
How would you like to configure ESLint?
と表示されるので「To check syntax, find problems, and enforce code style」を選択
ほかの上2つはルールが甘いので非推奨
? How would you like to use ESLint? To check syntax only To check syntax and find problems ❯ To check syntax, find problems, and enforce code style
次にWhat type of modules does your project use? と質問されるのでimportやrequireを使用しないなら「None of these」を選択
? What type of modules does your project use? JavaScript modules (import/export) CommonJS (require/exports) ❯ None of these
次にWhich framework does your project use? と質問されるのでReactやVueを使用していなければ「None of these」を選択
? Which framework does your project use? React Vue.js ❯ None of these
次にDoes your project use TypeScript? と質問されるのでTypeScriptを使用していなければNを入力してEnter
? Does your project use TypeScript? No
Where does your code run? は通常はBrowserを選択
? Where does your code run? ❯◉ Browser ◯ Node
How would you like to define a style for your project? はAnswer questions about your style を選択
? How would you like to define a style for your project? Use a popular style guide ❯ Answer questions about your style Inspect your JavaScript file(s)
What format do you want your config file to be in? と設定ファイルを質問されたらYAMLを選択
? What format do you want your config file to be in? (Use arrow keys) JavaScript ❯ YAML JSON
JavaScriptやJSONだと{}や,の付け忘れなどでエラーを発生させやすいためESLintに詳しくなければYAML推奨。
What style of indentation do you use? はSpacesを選択。最近はインデントでタブが使用されることは少ない。
? What style of indentation do you use? Tabs ❯ Spaces
What quotes do you use for strings? はSingleを選択。最近はシングルクォーテーションが主流。
? What quotes do you use for strings? Double ❯ Single
What line endings do you use? はMacならUnixを選択
? What line endings do you use? (Use arrow keys) ❯ Unix Windows
Do you require semicolons? はセミコロンを使わないならNを選択。
最近は記述量や可読性の観点から付けないことが多い。
? Do you require semicolons? No
以上の質問の回答が完了すると設定ファイル(.eslintrc.yml)が書き出される。
書き出された.eslintrc.ymlは次のようになっている。
indentがデフォルトだと4だが最近は2が主流。
YAML選択時の設定ファイル
env: browser: true es6: true extends: 'eslint:recommended' globals: Atomics: readonly SharedArrayBuffer: readonly parserOptions: ecmaVersion: 2018 rules: indent: - error - 4 linebreak-style: - error - unix quotes: - error - single semi: - error - never
eslint:recommendedについて
extends: 'eslint:recommended'はESLintの推奨ルールをまとめて設定している。
eslint:recommendedについてはESLintのRulesのページで確認できる。
チェックマークが付いているものが有効になっているものだ。
ESLint Rules
http://eslint.org/docs/rules/
例えばno-consoleはerrorが有効になっているのでconsoleがコード内に含まれるとエラーになる。
これをoffにしたい場合はrules:に
no-console: - off
を追加する
errorやoffは数字で指定することもできる
no-console: - 0
文字指定 | 数字指定 |
---|---|
off | 0 |
warn | 1 |
error | 2 |
実際に実行してみる
eslint test.js
のようにファイルを指定して実行する。
jsファイルすべての場合は*.jsのように指定。
VS CodeなどのエディタであればESLintの拡張機能をインストールすればJavaScriptを書いている最中に間違っている箇所を教えてくれる。
test.jsコード例
var arr = [7, ,9];
function add(a, b) {
var str;
var str = "Answer is ";
return str + a + b;;
}
console.log(add(1, 2));
eslint test.jsの結果はこうなる。
1:5 error 'arr' is defined but never used no-unused-vars 1:11 error Unexpected comma in middle of array no-sparse-arrays 5:9 error 'str' is already defined no-redeclare 5:15 error Strings must use singlequote quotes 6:3 error Expected indentation of 4 space characters but found 2 indent 6:22 error Unnecessary semicolon no-extra-semi 6:22 error Unreachable code no-unreachable 9:1 error Unexpected console statement no-console
エラーの内訳
no-unused-vars
使用されていない変数の宣言がある
no-sparse-arrays
[7, ,9]のように配列のカンマ内がカラ
no-redeclare
変数が2回以上宣言されている
quotes
singleが指定されているのにダブルクォーテーションが使用されている
indent
インデントに4が使用されているのに2になっている
no-extra-semi
b;;のように不要なセミコロンがある
no-unreachable
return;のあとに記述がある
このコードだとreturn str + a + b;;になっているため、このエラーが表示される。
no-console
コード内にconsoleが含まれている。
つまり、このように修正すればエラーは発生しなくなる。
※no-consoleはoffに設定
var arr = [7, 9];
function add(a, b) {
var str = 'Answer is ';
return str + a + b;
}
console.log(arr);
console.log(add(1, 2));